
def set_debug_mode(self, debug_mode:bool): This function sets the debug root logger to be at DEBUG level.If in future you wish you rework this class to accommodate for more handler management, be careful of making duplicate handlers. If it so happens that in your application you have to recreate a child logger, this function will stop duplicate handlers. def add_handlers(): the function add handlers checks if it's not duplicating handlers.
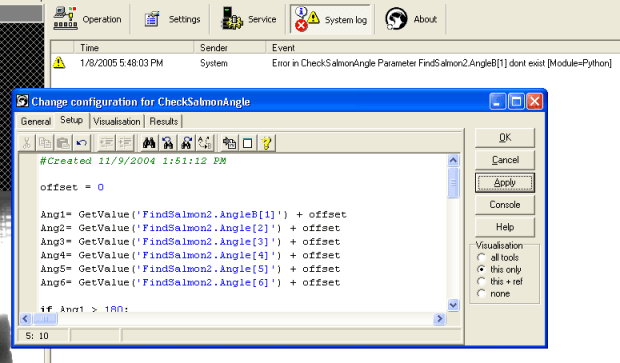
In future, you can use this singleton class to also handle handlers. Handlers: here we create 2 handlers, a terminal output and a file handler.def get_logger(self, logger_name): This is the function we will call in all the modules to initiate a child logger.Since this is a logger, we don’t need to be reinstantiating anyway. Otherwise, it will return the same instance. If it is, it will instantiate an instance. def _new_(cls): This is the part that makes this class a singleton, it checks if the instance is none.The functions are self-explanatory with the docstrings but it's important to note a couple of important elements. For the “debug mode” we speak of, it’s not required. I find it much more versatile to have a global single instance class a centralised access point for child loggers. Or it could be used to redefine the log after an attribute of the class is changed. I’ve left it a singleton class for future implementations, for example, it could serve as a global class which holds all the child loggers as attributes. So every time we start a child logger class, it follows the same framework we define. The reason we use it here is to have a global logger class with the features we want. Here’s a really good explanation:Ĭlick on here to go to Geek for Geek's explanation You can access and change variables of this instance throughout your program in any python module without having to instantiate the class again.įor the specifics of singleton classes and why they work, I won’t reinvent the wheel. That single class cannot be instantiated again. Imagine a class object that you instantiate once. This one instance remains throughout the lifetime of a program. Just make sure you modify the framework accordingly.įor those asking: What is a singleton class?Ī singleton class in python is one that allows you to create one instance of a class. You can get away with just defining the functions without the class. Like I said earlier, this does not have to be a class, nor does it have to be a singleton class in particular. This is located in the Logger folder in logger.py. Optional: put a tests folder and test the logger features here.Put your application modules within my_app or any other folder your modules will be.Create a main.py being your main run file.Have a logger.py inside your logger folder.Have a folder called my_app (or your application name) and make sure there’s an _init_.py.Have a folder called logger, and make sure there’s an _init_.py.Which in most cases, and our case is the ROOT logger. Info, debug, warning etc.) then it will inherit the parent class’ setting. When a child logger does not have a logging level specified (i.e. It is known to be best practice that you create a child class for every module. If the bracket is empty, then it is the root logger. Whenever you initiate logging.getLogger(_name_) you create a CHILD logger.The first one I find to be the most versatile during a running application, and so this is the framework I follow There are multiple ways to configure loggers: writing functions/classes, dictConfig, using logging.basicConfig, a config YAML file etc.There are, however, a couple of concepts I need to touch on that are relevant: I wouldn’t want to reinvent the wheel so here’s a link to the documentation:Īnd a link to a good explanation of loggers.

I am going to assume you have some basic knowledge of the python logging package. I won't go into explaining the concept of the Python logging package. Required Background information on Loggers He’s on the right track, but still, that doesn’t help give us the option to change aspects of all logging on the fly.
